Set up SDK
This page gives information about how to set up TentuPlay SDK to make an in-app message work. You will learn the following:
-
The prerequisites that are required
-
Controllers, classes, and methods that are applicable
-
How to configure SDK in Unity editor
-
UI components and their scripts for offers
Prerequisites
This page assumes that you are finished with the following:
-
You have created a TentuPlay account and a project in console
-
You have downloaded and installed TentuPlay SDK v2021.2 or later
-
You have completed common TentuPlay Unity SDK setup and configuration for data collection and upload
Controller, class, and methods in use
When ShowOfferById
or ShowLatestOffer
is called, TPPersonalizedOfferController will dynamically retrieve offers by player (namely, player_uuid
) and show them on Scene.
-
ShowOfferById
andShowLatestOffer
are methods of theTPPersonalizedOffer
class -
TPPersonalizedOffer
is a class for displaying TentuPlay in-app messages and AI In-Game Shop offers
These components (namely, controller, class, and methods) are used commonly by both in-app messages and AI in-game shop offers. |
Set up in Unity editor
To make a message appear correctly, you need to work on both Unity editor and your script.
-
Locate TPPersonalizedOfferController.prefab in Assets/TentuPlay/TPPersonalizedOfferTemplates in Unity editor. It contains its script (TPPersonalizedOfferController.cs) as a component.
-
Add TPPersonalizedOfferController game object to Scene.
-
Call
ShowOfferById
orShowLatestOffer
by usingtpPersonalizedOfferController
GameObject parameter value as shown in the code example.Code examplepublic void ShowPersonalizedOffer() { TPPersonalizedOffer myTPOffer = new TPPersonalizedOffer(); StartCoroutine( myTPOffer.ShowOfferById(tpPersonalizedOfferController, player_uuid, "en", offer_id, (response => { }))); }
For more examples, check out Assets/ShingGoongDemo/Scripts/TentuplayRelated/TentuPlayCRMPlayerController.cs and Assets/ShingGoongDemo/Scripts/TentuplayRelated/MailBoxOpen_offer.cs.
-
Now, an example message appears on on the game screen when it is meant to be shown.
In-app message on mail boxIn-app message message when opened
The OfferInfo.offerType is the attribute that differentiates between in-app messages and AI in-game shop offers |
Message UI
Let’s take a brief look at what the UI components of in-app message are and how they are organized in Unity.
UI templates
When ShowOfferById
or ShowLatestOffer
is called, either TentuPlayManualOffer_landscape or TentuPlayManualOffer_portrait is generated on Scene depending on screen orientation as a child of TPPersonalizedOfferController (TPPersonalizedOfferController.ShowOffer
).
The image entered via the console becomes a Texture/Raw Image of OfferImage. |
.png)
UI template components
For instance, let’s learn more about TentuPlayManualOffer_landscape.prefab that is one of two assets that contain message UI elements.
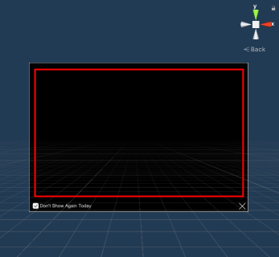
There are two scripts inside TentuPlayManualOffer_landscape.prefab.
-
MessageController: A script to open and close messages
-
TentuPlayManualOffer: A TentuPlay script for in-app messages
Message image (OfferImage
)
GUI | Scripts | ||
---|---|---|---|
|
TentuPlayOffer.GoToOfferEvent in On Click() |
Verify messages
For security reasons, TentuPlay provides a verification API that enables you to authenticate the offer’s identity and check its integrity without any forgery and corruption.
To learn how to use the verfication API, see Offer verification API.